Before start using Stripe in your application, create an account in stripe and get your secret API key.
- Install the
stripe
npm package: - Import stripe:
Create a Billing Portal Session
Purpose: The Stripe Billing Portal is a hosted customer portal that allows businesses to offer a self-serve experience for their customers. It’s particularly useful for managing subscriptions, invoices, and billing details
First you need to create a customer portal settings to create a portal session for customers.
You will face the below error if you try to create a portal session before save your customer portal settings in stripe.
StripeInvalidRequestError: You can’t create a portal session in test mode until you save your customer portal settings in test mode at https://dashboard.stripe.com/test/settings/billing/portal.
Follow below steps to create a customer portal settings in stripe
Customer portal
- Click on the link below to access the billing portal. https://dashboard.stripe.com/test/settings/billing/portal

“Key Features: You can customize invoices, customer information, payment methods, cancellations, subscriptions, and business information.”
Refer this document provided by stripe to allow your customers to self-manage their payment details, invoices, and subscriptions in one place https://stripe.com/docs/customer-management
2. Create a billing portal session
app.get(“/billing_session_url”, async (req, res) => {
try {
const session = await stripe.billingPortal.sessions.create({
customer: #YOUR_CUSTOMER_ID#,
return_url: ‘http://localhost:4200/payment’,
});
if (session) {
res.status(200).json({
status: 200,
message: ‘stripe billing portal session created successfully.’,
data: session,
});
}
} catch (error) {
console.log(error)
}
});
3. Below is the response you received from the above API:
{
“status”: 200,
“message”: “stripe billing session created successfully.”,
“data”: {
“id”: “bps_1OFEjxB….”,
“object”: “billing_portal.session”,
“configuration”: “bpc_1OFEhzBqz…”,
“created”: 1700653029,
“customer”: #YOUR_CUSTOMER_ID#,
“flow”: null,
“livemode”: false,
“locale”: null,
“on_behalf_of”: null,
“return_url”: “http://localhost:4200/payment”,
“url”: “https://billing.stripe.com/p/session/test_YWNjdF8xSEg4Q2pCcWJSSnVWRjlNLF9QM0xRZ1lrTGdSaT……”
}
}
4. You will land on the page below using the link you received from Stripe.
4.1) Currently, the customer is on a monthly subscription
4.2) Customers can update their plan from monthly to yearly.

5. Cancel your current subscription plan
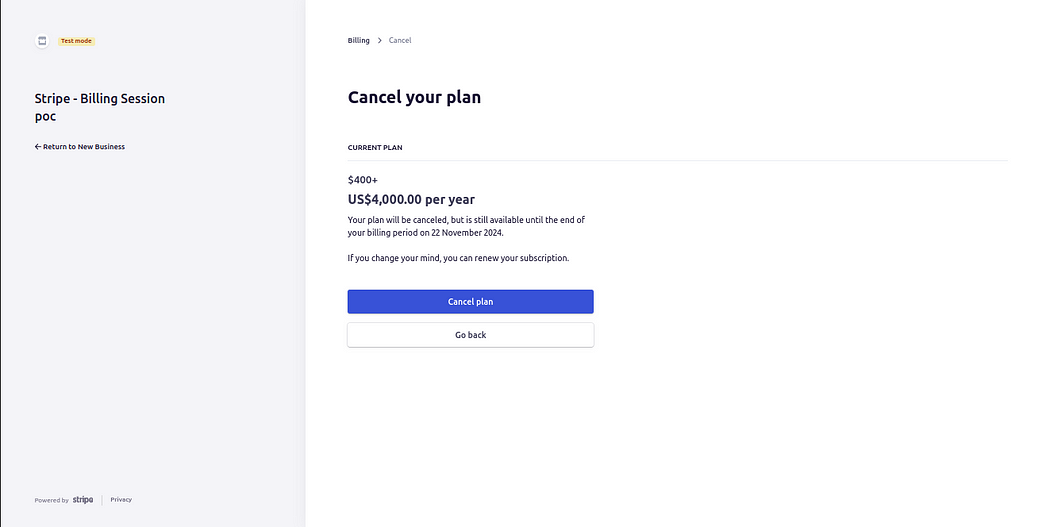
Checkout Session
Purpose: Checkout Session is a tool provided by Stripe to create a streamlined and customizable checkout experience for one-time purchases or product sales.
- Create a checkout session via API
try {
const paymentMethods = await stripe.paymentMethods.list({
“customer”: #YOUR_CUSTOMER_ID#,
type: ‘card’,
});
const session = await stripe.checkout.sessions.create({
payment_method_types: [paymentMethods.data.card],
mode: ‘subscription’,
line_items: [
{
price: #REPLACE_PLAN_ID_FROM_STRIPE#,
quantity: 1,
},
],
customer: #YOUR_CUSTOMER_ID#,
success_url: ‘http://localhost:4200/plans?customerId=YOUR_CUSTOMER_ID’,
cancel_url: ‘http://localhost:4200/plans’,
});
res.send({
status: 200,
data: session,
});
} catch (error) {
console.log(error);
res.status(400).send(`checkout Error: ${error.message}`);
}
});
2. The response you received from the above code
{
"status": 200,
"data": {
"id": "cs_test_a1hJ7sO0MGOEJtwYEB6bNHF....",
"object": "checkout.session",
"after_expiration": null,
"allow_promotion_codes": null,
"amount_subtotal": 40000,
"amount_total": 40000,
"automatic_tax": { "enabled": false, "status": null },
"billing_address_collection": null,
"cancel_url": "http://localhost:4200/plans",
"client_reference_id": null,
"client_secret": null,
"consent": null,
"consent_collection": null,
"created": 1700660602,
"currency": "usd",
"currency_conversion": null,
"custom_fields": [],
"custom_text": {
"shipping_address": null,
"submit": null,
"terms_of_service_acceptance": null
},
"customer": #CUSTOMER_ID#,
"customer_creation": null,
"customer_details": {
"address": null,
"email": "xyz@yopmail.com",
"name": null,
"phone": null,
"tax_exempt": "none",
"tax_ids": null
},
"customer_email": null,
"expires_at": 1700747002,
"invoice": null,
"invoice_creation": null,
"livemode": false,
"locale": null,
"metadata": {},
"mode": "subscription",
"payment_intent": null,
"payment_link": null,
"payment_method_collection": "always",
"payment_method_configuration_details": null,
"payment_method_options": null,
"payment_method_types": ["card"],
"payment_status": "unpaid",
"phone_number_collection": { "enabled": false },
"recovered_from": null,
"setup_intent": null,
"shipping_address_collection": null,
"shipping_cost": null,
"shipping_details": null,
"shipping_options": [],
"status": "open",
"submit_type": null,
"subscription": null,
"success_url": "http://localhost:4200/plans?customerId=CUSTOMER_ID",
"total_details": {
"amount_discount": 0,
"amount_shipping": 0,
"amount_tax": 0
},
"ui_mode": "hosted",
"url": "https://checkout.stripe.com/c/pay/cs_test_a1hJ7sO0MGOEJtwYEB6bNHF..."
}
}
3. You will land on the page below using the link you received from Stripe
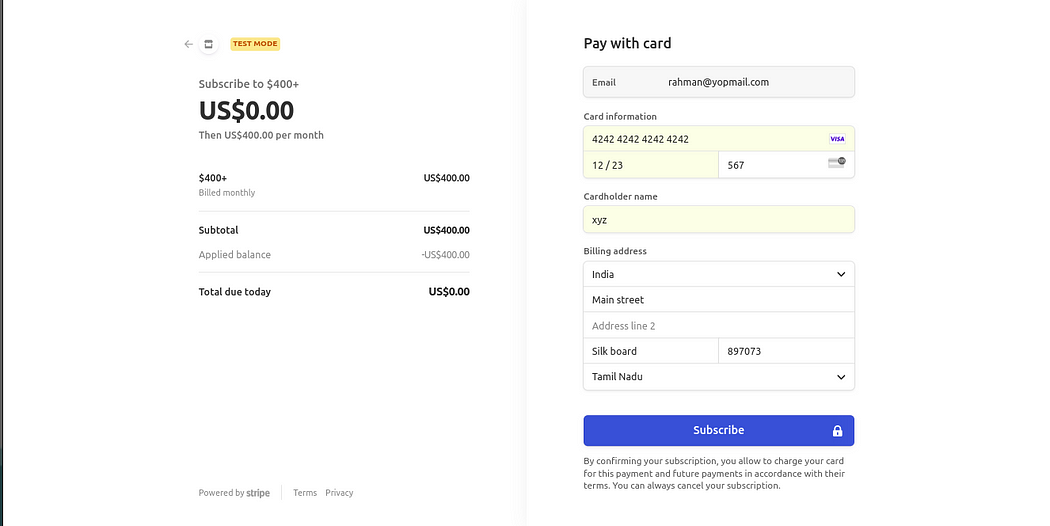
4. In Stripe, webhooks play a crucial role in keeping your application or system informed about events that occur on your Stripe account. Webhooks enable you to receive real-time notifications about various activities, such as successful payments, failed payments, subscription changes, and more.
“Stripe webhook link: https://dashboard.stripe.com/test/webhooks“
4.1) “Use Stripe CLI to simulate Stripe events in your local environment: https://dashboard.stripe.com/test/webhooks/create?endpoint_location=local“
app.use((req, res, next) => {
if (req.originalUrl.startsWith(‘/payment/webhook’)) {
express.raw({ type: ‘application/json’ })(req, res, next);
} else {
next();
}
});
const endpointSecret = process.env.STRIPE_WEBHOOK_ENDPOINT_SECRET;
const sig = req.headers[‘stripe-signature’];
const event = stripe.webhooks.constructEvent(req.body, sig, endpointSecret);
//You can process the event data based on your needs//
Conclusion:
Both the Billing Portal and Checkout Session are part of Stripe’s efforts to simplify the complexities of online payments, subscriptions, and billing for businesses, providing a secure and efficient way to handle customer transactions. Businesses can leverage these tools to enhance the user experience, reduce friction in the payment process, and manage subscriptions and invoices with ease.